As you may have noticed, Firefox for desktop computers (Windows, Mac, and Linux) got a redesigned interface with the release of Firefox 29.0. This redesigned browser called for redesigned web pages to showcase the new interface (the tabs, icons, menus, etc., collectively called “browser chrome”) and new features (especially the new customization menu)
Naturally, the main audience for these pages are people using browsers that aren’t Firefox, so we wanted to illustrate the new Firefox design in a fun and compelling way that gives them a real sense of what it looks like, hopefully encouraging folks to download it and see it first hand. Another big target audience are Firefox users who haven’t yet updated, so we needed to give them an overview of what’s new.
This also gave us a chance to create some snazzy animations to show off some of the advances in CSS and SVG supported by the current generation of browsers, both on desktop computers and mobile devices. Here’s how we made it.
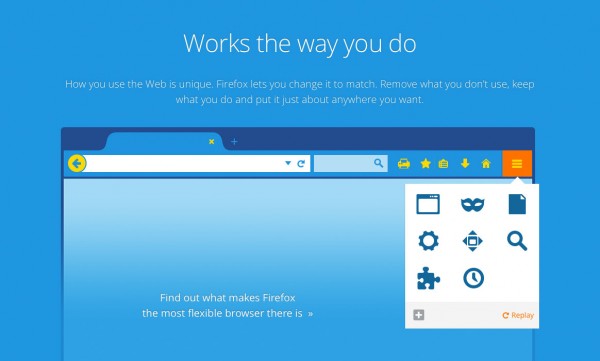
Browser Chrome Animations
In order to demonstrate features of Firefox, we needed to simulate the way interface elements respond to user actions; opening and closing tabs, rearranging icons, adding a bookmark, and so on. This called for fairly complicated animation sequences that had to be quick and buttery smooth. The complex interplay of multiple elements moving both sequentially and in tandem really drove home the need for a CSS animation editor (vote it up!).
Approach & structure
Each animation illustrating the browser chrome (One, Two, Three) is wrapped in a div
element with a common class applied (animation-wrapper
), along with an id
we can use to target the specific element with JavaScript.
The first element inside the animation wrapper is a composite fallback image for browsers that don’t support CSS animations. This image has a common classname (fallback
) for easy CSS targeting. We can conditionally hide this image by leveraging the cssanimations
class Modernizr applies to the body
. By first assuming that animation is not supported, we ensure a functional degradation for even the oldest and least capable browsers, and we can progressively enhance the page for more advanced browsers that support the more advanced features.
The next element inside the wrapper div
is the stage for the entire animation – <div class="stage">
, really just an invisible box in which other elements can move around. Using the same cssanimations
class from Modernizr, we’ll display the stage for browsers that can handle the animation.
/* for legacy browsers */
.fallback {
display: block;
}
.stage {
display: none;
}
/* for modern browsers */
.cssanimations {
.fallback {
display: none;
}
.stage {
display: block;
}
} |
/* for legacy browsers */
.fallback {
display: block;
}
.stage {
display: none;
}
/* for modern browsers */
.cssanimations {
.fallback {
display: none;
}
.stage {
display: block;
}
}
(We use Less to preprocess our CSS, so those nested rules are converted into separate rules with descendant selectors.)
The final task is to trigger the animations only when they come into view, as there’s no sense running an animation while it’s off screen. We used jQuery Waypoints to monitor the page’s scroll position, adding an animate
class to each wrapper div
when it comes into view. The addition of that class sets off the CSS animation sequence.
.animating-element {
position: absolute;
top: 10px;
right: 40px;
}
/* animate class added via JavaScript/Waypoints based on scroll position */
.animate {
.animating-element {
animation: moveAround 0.7s ease 0s 1 normal forwards;
}
} |
.animating-element {
position: absolute;
top: 10px;
right: 40px;
}
/* animate class added via JavaScript/Waypoints based on scroll position */
.animate {
.animating-element {
animation: moveAround 0.7s ease 0s 1 normal forwards;
}
}
This approach worked well and helped us keep each animation block self-contained and modular. It provided a common and easily customizable HTML & CSS structure for each animation, and less capable browsers still have access to all the content in a well styled page. Within that stage box we can add any other content or elements we need.
Timing is everything
The browser chrome animations have multiple elements with multiple animations applied, so getting the timing just right became rather tedious. Because separate animations are completely independent in CSS, there’s no simple way to tell a browser to “start animationY 2.1 seconds after animationX completes.” Instead, you need to do the calculations yourself and hard code them into each animation declared in the CSS, liberally using animation-duration
and animation-delay
to fire off each step of the scene in sequence. The mental gymnastics go something like this:
Step 1 has a 0.7 second delay and runs for 1.5 seconds. Then Step 2 should start 1.4 seconds after Step 1 completes, so it should have a delay of… 3.6 seconds. Step 2 runs for 2 seconds, and Step 3 needs to begin a quarter of a second before Step 2 completes, so Step 3 needs a delay of 5.35 seconds…
As you can imagine, the more elements you animate and the more steps you have in the sequence, the harder the math becomes. Adjusting the timing of one step in the chain can mean adjusting all the subsequent steps to compensate.
Designer Ty Flanagan created video mockups in Adobe After Effects to serve as a guide for the CSS animation, which was an enormous help. There was still a fair amount of fine tuning to be done by hand, constantly refreshing the page and tweaking a few milliseconds until it just “felt right,” but that process could have taken much longer without the videos for reference.
Another way to do all of this would have been controlling the chained animations in JavaScript, relying on the animationend
event to fire off the next step in the sequence. However, a bunch of event listeners and setTimeout
calls in a script probably wouldn’t have been a faster or better approach.
Animations in a Circle
Some of our favorite animations are the customize icons, mostly because the circular mask effect is so neat in its simplicity.
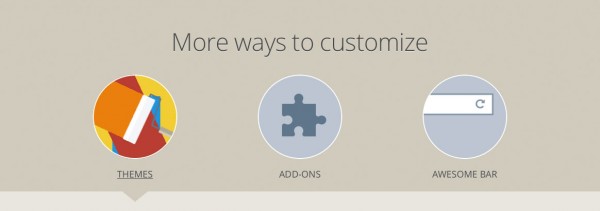
The key to achieving the circular mask is a bit of absolute positioning and the incredibly versatile border-radius
. The markup isn’t too complex – a stage to contain everything, a div
for the circular mask, and whatever elements need to be animated.
If you’d like to see an example and play around with the code before reading about the methodology, here’s a little demo on CodePen.
The stage
The stage has a set height and width with a hidden overflow and relative positioning. The background color of the stage fills the circular mask.
.stage {
position: relative;
width: 300px;
height: 180px;
overflow: hidden;
background: #fff;
} |
.stage {
position: relative;
width: 300px;
height: 180px;
overflow: hidden;
background: #fff;
}
The circular mask
The circular mask is absolutely positioned at the center of the stage, calculated by (stage width - (mask width + mask border width))/2
(this equation could be simpler with box-sizing: border-box
). The mask has a wide enough border to reach just past the furthest boundary of the stage. The border bumping up against the page background is what completes the illusion of the mask, so the mask’s border color matches that of the section’s background color (sadly, this means the technique only works with a solid colored background).
To make sure the mask covers the animated elements, it has a z-index
at least one higher than the front-most animated element.
.circular-mask {
position: absolute;
width: 164px;
height: 164px;
border: 100px solid #ccc;
border-radius: 50%;
top: -100px;
left: -32px;
z-index: 2;
}
/* animated elements share absolute positioning and
a z-index lower than .circular-mask */
.animated {
position: absolute;
z-index: 1;
} |
.circular-mask {
position: absolute;
width: 164px;
height: 164px;
border: 100px solid #ccc;
border-radius: 50%;
top: -100px;
left: -32px;
z-index: 2;
}
/* animated elements share absolute positioning and
a z-index lower than .circular-mask */
.animated {
position: absolute;
z-index: 1;
}
The animated elements
The only requirement for the animated elements is that they reside inside the stage and have a z-index
lower than the mask. Otherwise, anything goes.
Though purely flair (as opposed to the feature demonstrations provided by the browser chrome animations), these circular animations were fun to build and we’re very pleased with the result.
Drawing Firefox in the browser
When we first watched a video mockup of the proposed intro animation for the new Firefox for Desktop landing page, we wondered if this was actually possible to pull off in a web browser. The animation involves a series of moving lines which fill in as the outlines fade onto the web page, creating an illustrated image of the Firefox browser. Definitely not a typical animation you see on the web every day!
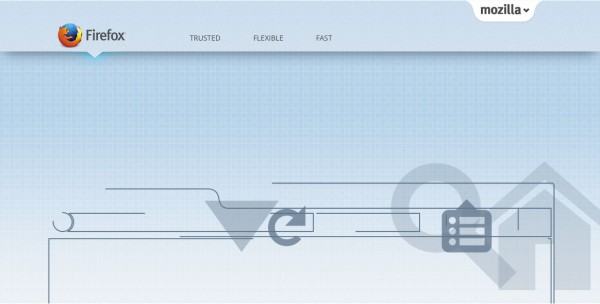
The first step on the path of discovery was to choose an appropriate image format. SVG seemed like the most obvious choice, given that the images needed to scale. Nobody on the team had any prior experience with SVG animation but it seemed like a fun challenge! Ty came up with a rough demo showing how we might use SVG path strokes for the moving lines, which seemed like a perfect starting point. We could have chosen to use an SVG animation library such Raphael or SnapSVG, but we wanted to try to keep our dependencies as light as possible (we had plenty already; no reason to add any more if we can avoid it). The timing and intricacies of the animation made a strong case for trying to use CSS keyframe animations, and this would also be a good opportunity to show off their potential. It was then we recalled this really clever technique that could pull off the same line-drawn effect using CSS.
Animating SVG line paths using CSS
The trick to the line drawing effect is to animate the stroke-dashoffset
of an SVG image path. The stroke-dasharray
property allows you to apply a dashed border effect to the outline of an SVG image. The clever part is that if you set the length of the dash equal to the total length of the image path, you can then animate stroke-dashoffset
to make it appear as if the line is being drawn one segment at a time. Magic!
Here’s an example of an SVG path:
And some CSS to animate it:
.circle {
stroke-dasharray: 117;
stroke-dashoffset: 117;
animation: draw-circle 5s linear forwards;
}
@keyframes draw-circle {
100% {
stroke-dashoffset: 0;
}
} |
.circle {
stroke-dasharray: 117;
stroke-dashoffset: 117;
animation: draw-circle 5s linear forwards;
}
@keyframes draw-circle {
100% {
stroke-dashoffset: 0;
}
}
You can find the required length of a path pretty easily using a bit of JavaScript:
var circle = document.querySelector('.circle');
var length = circle.getTotalLength(); |
var circle = document.querySelector('.circle');
var length = circle.getTotalLength();
The animation on the finished page is quite a bit more complicated than this example, but hopefully you can get the idea. We also animated fill-opacity
and stroke-opacity
to color in the browser panels and fade out the lines at the end of the animation, leaving a scalable vector drawing of the new Firefox.
Scaling SVG using CSS transforms
As well as animating the line drawing, we also needed to scale the image as it zooms onto the page. From there, the icons also zoom into their appropriate places. This was all done using regular CSS transforms via translate
and scale
.
There are some notable cross-browser inconsistencies here when it comes to scaling SVG using this method. Both Chrome and Safari render a bitmap of an SVG prior to performing a CSS transform. This is presumably for performance reasons, but it does lead to blurry images when you blow them up. Firefox seems to weigh up performance and image quality a little differently, and renders sharper images when they scale. To get around the resizing issues, the best solution was to render icons at their largest size initially and then scale them down, as opposed to the other way around. It seems browsers still have some work to do in this area in order to improve SVG rendering under these circumstances.
Putting it all together
Combining all the separate CSS keyframe animations together was probably the most time consuming task. We can also look forward to the day when we no longer need vendor prefixes for CSS keyframe animations, as the duplication of code required is still a bit undesirable. Aside from this, getting the timing right was once again the trickiest part. For a less-than-5-second animation, having to reload and run through the whole sequence over and over made the process pretty time consuming (here’s another vote for that CSS animation editor).
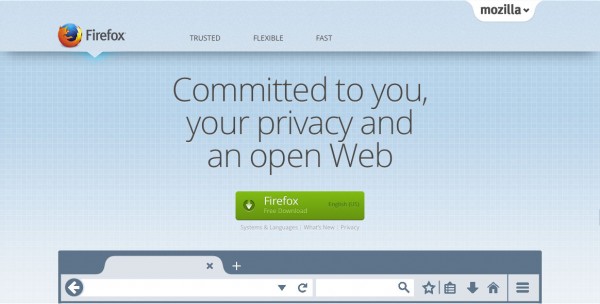
The final result of all this work is a set of pages that beautifully show off what the new desktop Firefox looks like while also showing off what it can do with open web technologies. If you haven’t yet, please do check it out. It’s all responsive, mobile-friendly, progressively enhanced, retina-ready, and still pretty light weight all things considered. And without a single byte of Flash.
The team
- Jon Petto – Developer
- Alex Gibson – Developer
- Holly Habstritt Gaal – UX Designer
- Ty Flanagan – Graphic Designer
- Matej Novak – Copywriter
- Jennifer Bertsch – mozilla.org Product Manager
- Mike Alexis – Program Manager
This article was co-written by Jon Petto and Alex Gibson, with editorial assistance from Craig Cook.